[Pandas] Indexing, Selecting & Assigning
Native accessors
객체 접근법
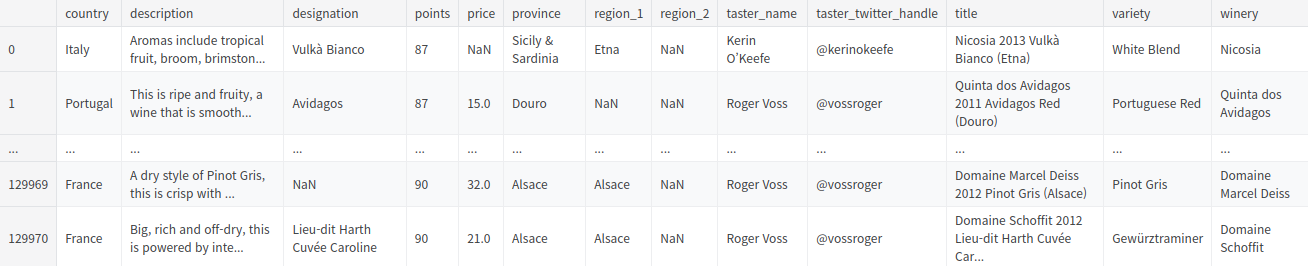
다음과 같은 DataFrame에서 속성에 대해 접근해보자
우리가 Country라는 속성에 대해 속성값을 조회하고 싶다면 다음과 같이 조회하면 된다.
reviews.country
0 Italy
1 Portugal
...
129969 France
129970 France
Name: country, Length: 129971, dtype: object
인덱싱 연산자 접근법
또한, 객체 접근법이 아닌 흔히 리스트에서 쓰이는 인덱싱 방법으로도 DataFrame에 값들에 대해 조회를 해볼 수 있다.
reviews['country']
0 Italy
1 Portugal
...
129969 France
129970 France
Name: country, Length: 129971, dtype: object
reviews['country'][0]
'Italy'
Indexing in pandas (판다스에서 인덱싱)
Pandas에서 접근 방식이 이런 접근 방식뿐만 아니라 Pandas 자체 접근 연산자가 있다. 자체 접근법은 고급 연산을 하기 위해 필요한 방식이다.
.iloc
이 연산자는 인덱스 기반 선택이다.
즉, 데이터에서 숫자를 위치를 기준으로 데이터를 선택한다.
행을 조회하는 방법
reviews.iloc[0]
country Italy
description Aromas include tropical fruit, broom, brimston...
...
variety White Blend
winery Nicosia
Name: 0, Length: 13, dtype: object
열을 조회하는 방법
첫 번째 행의 모든 열
reviews.iloc[:, 0]
0 Italy
1 Portugal
...
129969 France
129970 France
Name: country, Length: 129971, dtype: object
첫 번째 행에서 상위 3개의 열
reviews.iloc[:3, 0]
0 Italy
1 Portugal
2 US
Name: country, dtype: object
리스트로 열 조회하기
reviews.iloc[[0, 1, 2], 0]
0 Italy
1 Portugal
2 US
Name: country, dtype: object
.loc
loc
속성은 label 기반으로 하는 선택이다. 따라서, iloc 속성과 달리 조금은 인간에게 읽기 편한 방식으로 조회가 가능하고 실제로 이 방법을 제일 많이 쓴다.
country 속성에 첫 열
reviews.loc[0, 'country']
'Italy'
taster_name
,taster_twitter_handle
,point
속성에 있는 모든 열
reviews.loc[:, ['taster_name', 'taster_twitter_handle', 'points']]
taster_name | taster_twitter_handle | points | |
0 | Kerin O’Keefe | @kerinokeefe | 87 |
1 | Roger Voss | @vossroger | 87 |
... | ... | ... | ... |
129969 | Roger Voss | @vossroger | 90 |
129970 | Roger Voss | @vossroger | 90 |
.iloc 🤜🤛 .loc
loc
데이터프레임의 행이나 컬럼에 label이나 boolean array로 접근.
location의 약어로, 인간이 읽을 수 있는 label 값으로 데이터에 접근하는 것이다.
iloc
데이터프레임의 행이나 컬럼에 인덱스 값으로 접근.
integer location의 약어로, 컴퓨터가 읽을 수 있는 indexing 값으로 데이터에 접근하는 것이다.
Manipulating the index (인덱스 조작)
다음과 같이 만들어진 DataFrame에 인덱스를 추가할 수 있다.
reviews.set_index("title")
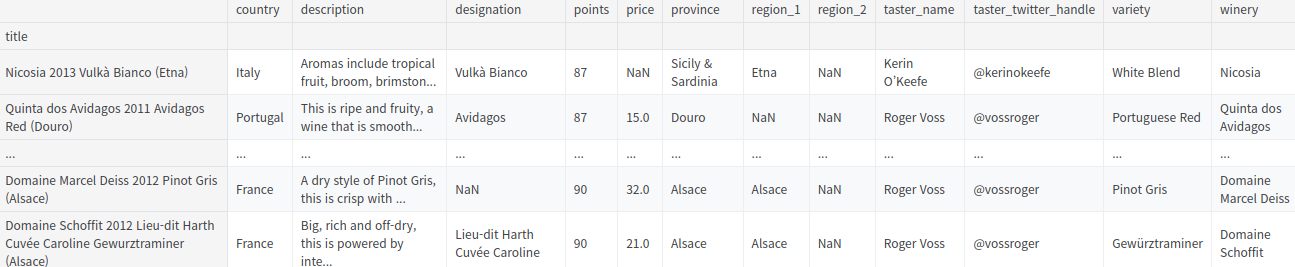
Conditional selection (조건부 선택)
앞서 배운 접근 법을 이용해서 특정 조건에 맞는 속성 값을 추출할 수 있는 방법이 있다.
객체 접근법을 사용한 조건부 선택
reviews.country == 'Italy'
0 True
1 False
...
129969 False
129970 False
Name: country, Length: 129971, dtype: bool
loc를 이용한 조건부 선택
reviews.loc[reviews.country == 'Italy']

reviews.loc[(reviews.country == 'Italy') & (reviews.points >= 90)]
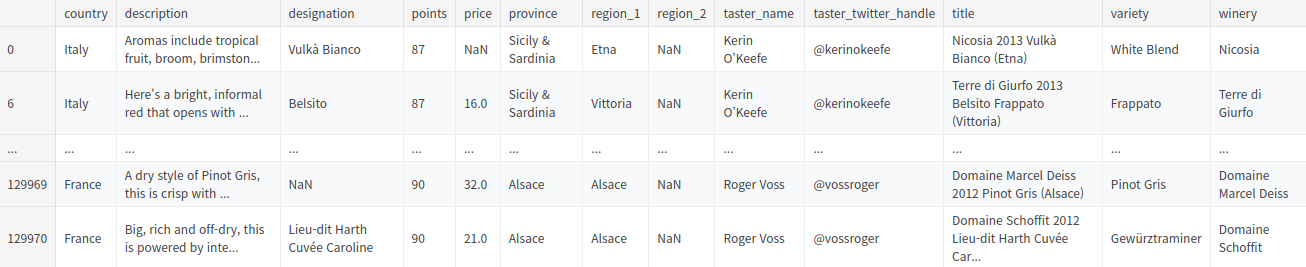
reviews.loc[reviews.country.isin(['Italy', 'France'])]

reviews.loc[reviews.price.notnull()]
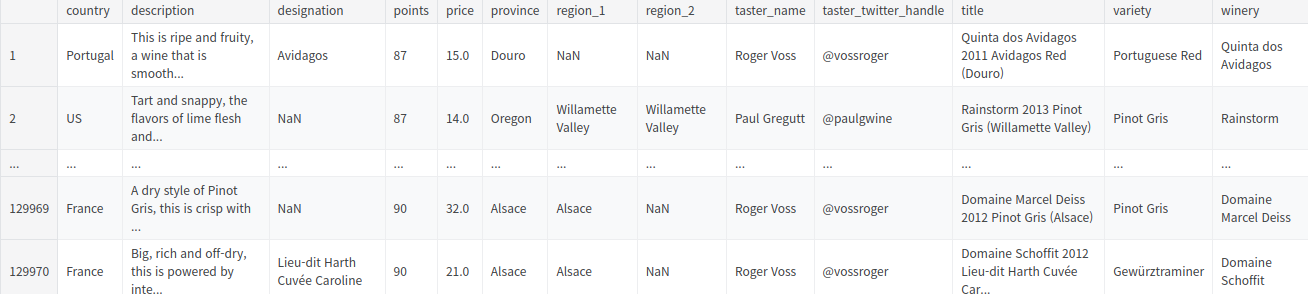