✍ 백준 26575 번 새천년관
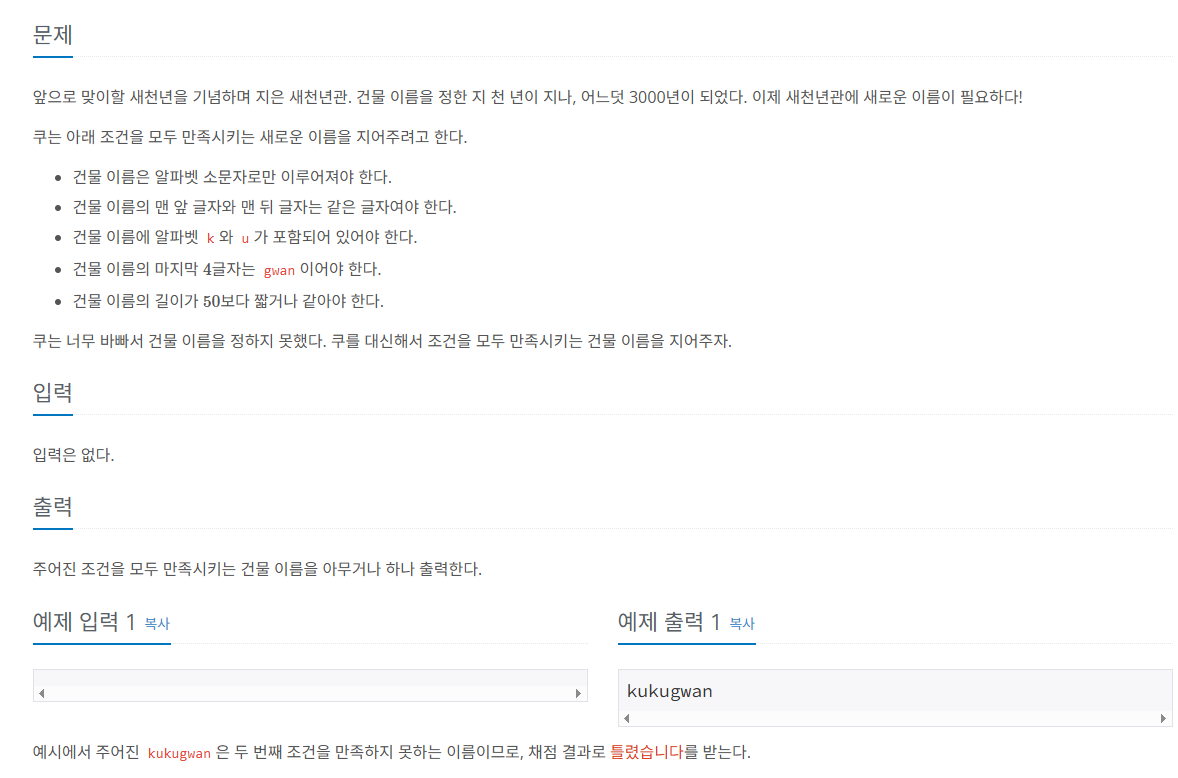
조건에 맞는 이름을 랜덤으로 생성해주는 문제이다.
랜덤함수가 있다는것은 알았지만, 랜덤에 대해 자세히는 몰랐기 때문에 랜덤함수에 대해 알아보기로 하였다.
📌 랜덤 값 생성
1. java.util.Random
클래스
먼저, 주요 메서드들은 이와 같다.
import java.util.Random;
Random rand = new Random();
// 0 이상 10 미만의 정수 (0~9)
int n = rand.nextInt(10);
// int 범위내의 무작위 정수
int m = rand.nextInt()
// long 범위내의 무작위 정수
int l = rand.nextLong()
// true 또는 false 무작위
boolean b = rand.nextBoolean();
// 0.0 이상 1.0 미만의 double
double d = rand.nextDouble();
// 배열에 무작위 바이트 생성
byte[] bytes = new byte[5];
rand.nextBytes(bytes)
이 랜덤 클래스가 만든 난수들은 사실 완전한 무작위가 아니라 어떤 규칙적인 계산을 통해 만들어진 값인데, 이 계산의 시작점을 시드 (Seed) 라고 한다.
Random rand1 = new Random(); // 자동 시드
Random rand2 = new Random(12345); // 지정 시드
Random r1 = new Random(123);
Random r2 = new Random(123);
System.out.println(r1.nextInt(100)); // 20
System.out.println(r1.nextInt(100)); // 32
System.out.println(r2.nextInt(100)); // 20
System.out.println(r2.nextInt(100)); // 32 시드값이 같아서 같은 결과가 나온다.
Random()
은 현재 시간을 기반으로 자동생성된다.시드를 이용하는 경우 같은 값이 생성되기 때문에, 디버깅등의 테스트에 유용하다.
2. Math.Random()
메서드
double r = Math.random(); // 0.0 이상 1.0 미만의 double 반환
// 0 이상 9 이하의 정수 만들기
int n = (int)(Math.random() * 10);
// 5~15 사이 정수 만들기
int min = 5;
int max = 15;
int randRange = (int)(Math.random() * (max - min + 1)) + min;
// 1.5 ~ 3.5 사이 실수 만들기
double min = 1.5;
double max = 3.5;
double randDouble = Math.random() * (max - min) + min;
Math.random()
메서드는 가장 간단하게 난수를 생성할 수 있는 메서드이다.0.0 ~ 1.0 사이의 실수만 주기 때문에, 우리가 원하는 범위로 바꿔줘야 한다.
3. 랜덤 활용 해보기
랜덤은 난수값이 숫자이기 때문에 랜덤 문자열은 인덱스로 접근해야 한다.
int length = 10; // 문자열 길이
String chars = "abcdefghijklmnopqrstuvwxyz";
StringBuilder sb = new StringBuilder();
Random rand = new Random();
// 랜덤 인덱스 생성
int index1 = rand.nextInt(chars.length()); // Random 클래스
int index2 = (int)(Math.random() * chars.length()); // Math.random() 메서드
for (int i = 0; i < length; i++) {
sb.append(chars.charAt(index1)); // 랜덤 문자 생성
}
System.out.println(sb.toString()); // 랜덤 문자열 생성
다음은 Random
클래스를 활용해 해결해본 코드이다.
import java.io.*;
import java.util.Random;
public class Main{
public static void main(String[] args) throws IOException {
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
Random random = new Random();
String allChars = "abcdefghijklmnopqrstuvwxyz";
String suffix = "gwan";
while (true) {
int totalLength = random.nextInt(45) + 5; // 총 길이 : 5~49 + gwan = 최대 50
int bodyLength = totalLength - suffix.length(); // gwan 제외한 앞부분 길이
StringBuilder sb = new StringBuilder();
sb.append('n'); // 첫 글자
boolean hasK = false;
boolean hasU = false;
for (int i = 1; i < bodyLength - 1; i++) {
char c = allChars.charAt(random.nextInt(allChars.length())); // 랜덤 글자 생성
if (c == 'k') hasK = true;
if (c == 'u') hasU = true;
sb.append(c);
}
sb.append('n'); // 마지막 글자 == 첫 글자
if (!hasK || !hasU) continue; // k와 u 없으면 다시 만들기
sb.append(suffix); // gwan 붙이기
String result = sb.toString();
bw.write(result);
break;
}
bw.flush();
bw.close();
}
}
📚마무리
랜덤 생성은 Random, Math.random() 이 있다.
Random 클래스의 시드는 같은 값이 생성되기 때문에 테스트에 유용하다.
문자열의 랜덤생성의 경우 인덱스의 랜덤값으로 접근한다.